Monolith to Microservices 1
This is a new series I’ve decided to write, which covers the current experiment I’m running with .NET Core 2.1, Azure DevOps, Docker and eventually Kubernetes.
I want to demonstrate how we break up a simplified example of a monolithic application containing self hosted WebAPI services into a number of microservices.
Code is hosted here:
https://github.com/r3adm3/monolithsvc and https://github.com/r3adm3/multiservice
The continuous integration pipelines are here :
https://techfrontier.visualstudio.com/dockerOrchestrationExperiment/_build?definitionId=22
Episode 1 then. Lets make a monolith.
Make sure on your chosen platform of choice you’ve got .NET SDK v5.0 installed.
Create a folder called monolithsvc and cd into it and then type:
This creates a boilerplate application ASP.NET Core MVC application. You can run it any time by doing:
By default the application will listen on port 5000. You should see something like this:
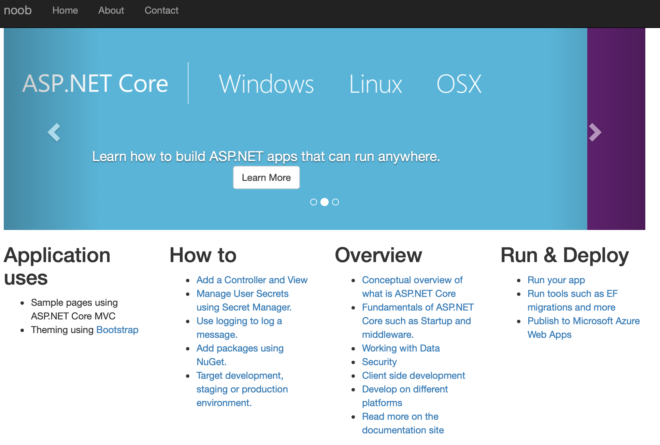
I’m not going to explain too much about Model-View-Controllers or WebAPI….but what we will do is create a number of controllers which will do some simple maths which we’ll change the front page to call into.
In the controllers folder create a new file called addController.cs, here’s the contents of mine:
1using System;
2using System.Collections.Generic;
3using System.Linq;
4using System.Threading.Tasks;
5using Microsoft.AspNetCore.Mvc;
6using monolithsvc.Models;
7
8namespace monolithsvc.Controllers
9{
10 [Route("api/[controller]")]
11 [ApiController]
12 public class addController : ControllerBase
13 {
14 // GET api/values
15 [HttpGet]
16 public ActionResult Get(int a, int b)
17 {
18 return (a+b);
19 }
20 }
21}
We can test this controller by launching the app with another “dotnet run” and then going to http://localhost:5000/api/add?a=2&b3
Now that’s the controller created, we need something to consume it. We’ll change the front page that has a form to collect two numbers and then use javascript to fetch the result from the controller.
Go in to Views > Home > Index.cshtml
1<table>
2<tbody>
3<tr>
4<td>First no:</td>
5<td><input id="a" type="text" /></td>
6</tr>
7<tr>
8<td>Second no:</td>
9<td><input id="b" type="text" /></td>
10</tr>
11</tbody>
12</table>
13<button>Add</button> <button>Minus</button> <button>Multiply</button>
14<div id="result"></div>
15
16@ViewBag.myFirstValue
17
18<script>
19 function operationFunction(op) {
20
21 var aNumber = document.getElementById("a").value
22 var bNumber = document.getElementById("b").value
23
24 if(op==='add'){var svcLink='api/add?'}
25 if(op==='minus'){var svcLink='api/minus?'}
26 if(op==='multiply'){var svcLink='api/multiply?'}
27
28 var req = new XMLHttpRequest();
29 req.open('GET', svcLink + 'a=' + aNumber + '&b=' +bNumber , true);
30
31 req.onload = function () {
32 if (req.status >= 200 && req.status < 400) {
33 console.log ('result ' + req.responseText);
34 var result = req.responseText;
35 document.getElementById("result").innerHTML = "Result " + result;
36 } else {
37 alert('Oh dear!');
38 }
39 }
40
41 req.send();
42
43 }
44
45
46
47</script>
…again we retest
I created controllers for taking away, adding and multiplying.
If you want to see the complete code at this stage it’s here
Next article we’ll talk about dockerising this application. http://www.techfrontier.co.uk/2019/03/02/building-up-some-microservices-breaking-up-the-monolith-2-dockerising-the-monolith/